Blackjack Suits
Black-Jack
Poker Suits Clip Art
This program is based on the following reading:Read at least the first half of this chapter before working on
the program below (practice questions at the bottom of this program).
publicclassBlackJack
{
publicstaticvoidmain(String[]args)
{
newBlackJack();
}
publicBlackJack()
{
Cardc1=randomCard();
Cardc2=randomCard();
printCard(c1);
printCard(c2);
if(sameCard(c1,c2))
{System.out.println('CHEATER!!!');}
if((c1.rank11&&c2.rank1)
(c1.rank1&&c2.rank11)
)
{System.out.println('BLACKJACK!!');}
}
classCard
{
intsuit,rank;
publicCard()
{
this.suit=0;this.rank=0;
}
publicCard(intsuit,intrank)
{
this.suit=suit;this.rank=rank;
}
}
publicCardrandomCard()
{
intsuit=(int)(Math.random()*4);
intrank=(int)(Math.random()*13+1);
Cardcard=newCard(suit,rank);
returncard;
}
publicvoidprintCard(Cardc)
{
String[]suits={'Clubs','Diamonds','Hearts','Spades'};
String[]ranks={'narf','Ace','2','3','4','5','6','7','8','9','10','Jack','Queen','King'};
System.out.println(ranks[c.rank]+' of '+suits[c.suit]);
}
publicbooleansameCard(Cardc1,Cardc2)
{
return(c1.suitc2.suit&&c1.rankc2.rank);
}
}
/******************************************************
This program uses some of the code from Chapter 11 of
the book 'Think Like a Computer Scientist'. The code
demonstrates sensible use of an INNER CLASS (Card).
The program is a basic start for a Black-Jack simulation.
- Black-Jack -
The rules of Black-Jack are:
(1) Each player receives 2 cards.
(2) The first player may take one more card, then one more,
trying to get as close to 21 total as possible.
(3) When the first player stops taking cards, the second
player takes cards to get closer to 21.
(4) If either player goes over 21, they lose immediately.
(5) If both players stay under 21, the player with the
higher total wins.
(6) At the beginning, if a player has 21 in two cards,
that is 'Black Jack' and they win immediately.
(7) The totals are added-up as follows:
Cards 2-10 count their face value (rank).
Face cards (king, queen, jack) count 10.
An ace counts either 11 or 1, depending on
which is better for the player.
Examples:
10 , 8 --> 18
Jack, Queen --> 20
Ace , 6 --> either 7 or 17
Queen , Ace --> Black-Jack (win)
Queen , 5 , 8 --> 23 (busted = lose)
= The Program =
The program above chooses 2 cards at random and prints them.
(0) Run the program lots of times until BLACKJACK is printed.
Also run it many times until it prints CHEATER.
(1) The check for BLACKJACK is wrong. It only says BLACKJACK
if a Jack and Ace are dealt. It must also accept
10 and Ace, Queen and Ace, or King and Ace.
Create a METHOD that checks for BlackJack and returns
a boolean TRUE or FALSE. It should accept 2 cards as parameters.
(2) Create a METHOD that returns the VALUE of a card, as follows:
2 --> 2 10 --> 10 Ace --> 11
3 --> 3 Jack --> 10
... Queen --> 10
9 --> 9 King --> 10
It must accept one CARD as a parameter. Do NOT use a number (rank)
as the parameter - give the entire card to the method.
(3) Create a METHOD called TOTAL that adds up the total value
of 2 Cards. It should call the VALUE method twice,
add the values, and return the answer.
(4) Create a new CLASS called HAND. This contains an ARRAY of CARDS.
The array should be big enough for 5 cards - more is not needed.
(5) Create a method called DEAL - this should choose 5 random cards,
and 'deal' these into a HAND. That means the HAND class
needs a CONSTRUCTOR that accepts 5 cards as parameters.
Then it must copy them into its array of 5 cards.

- It’s good to know these symbols if you’re unfamiliar, but in terms of Blackjack, the suit of cards are totally irrelevant. For each suit, there are nine numbered cards, 2 through 10, and four face cards, a King, Queen, Jack, and an Ace. That’s 13 cards per suit, but again, the suit isn’t important in Blackjack.
- Blackjack Basics. Premise of the Game. Blackjack is pretty simple. The basic premise of the game.
- Brybelly Classic Casino Dealer Armband 2-Pack - Authentic Elastic Blackjack Table Dealer Band Costume Accessory Set for Las Vegas Poker Game Night 5.0 out of 5 stars 2 $9.99 $ 9.
Pretty interesting! I really like playing Blackjack. However, I do not always prefer mobile versions, after all, I prefer online casinos as on casinotop. However, it all depends on taste. Someone better suited blackjack on Java. Blackjack Suit ‘Em Up Rules To start the game, the player selects the desired stake for each hand being played, and also a side bet for each hand if required. The side bet is optional. You do not have to play it on every hand, or even at all.
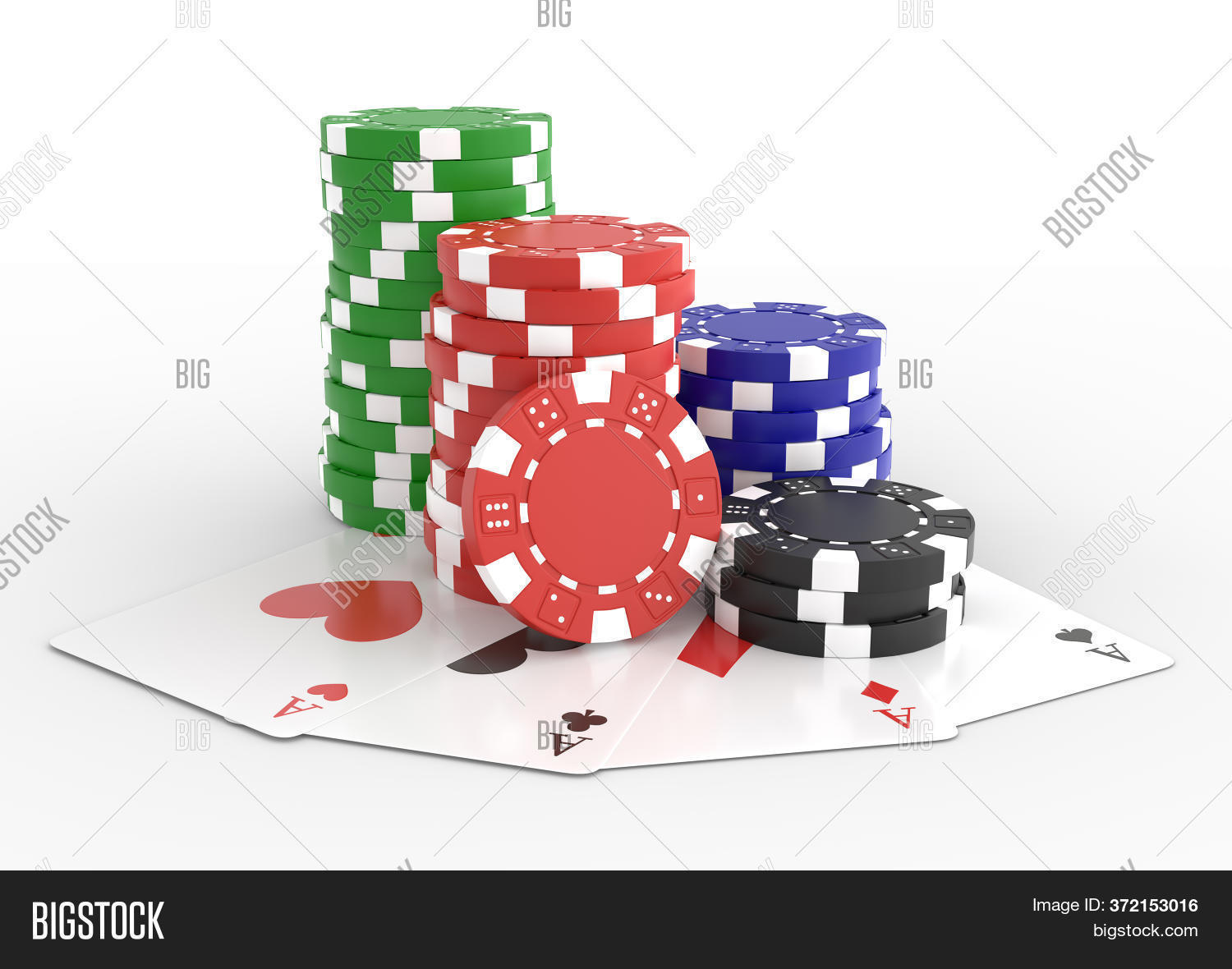
(6) Create a method called PRINTHAND to print out 5 cards.
It should accept a HAND as a parameter.
(7) In POKER, a HAND has 5 cards. If ALL 5 cards are the same suit
(5 hearts or 5 spades, etc) that is called a FLUSH. That is
a very good hand. Write a method called CHECKFLUSH that
accepts a HAND as a parameter, and checks whether all 5 cards
have the same suit. Then it returns TRUE or FALSE.
*******************************************************/
Solutions
publicbooleancheckBlackJack(Cardfirst,Cardsecond){
if((first.rank>=10&&second.rank1)
(first.rank1&&second.rank>=10))
{returntrue;}
else
{returnfalse;}
}
publicintvalue(Cardc)
{if(c.rank>10)
{return10;}
elseif(c.rank1)
{return11;}
else
{returnc.rank;}
}
publicinttotal(Cardfirst,Cardsecond)
{intv1=value(first);
intv2=value(second);
returnv1+v2;
}
classHand
{
Card[]cards=newCard[5];
publicHand(Cardfirst,Cardsecond)
{
cards[0]=first;
cards[1]=second;
}
publicHand(Cardc0,Cardc1,Cardc2,Cardc3,Cardc4)
{
cards[0]=c0;
cards[1]=c1;
cards[2]=c2;
cards[3]=c3;
cards[4]=c4;
}
}
publicHanddeal()
{
Handthese=newHand(randomCard(),randomCard(),
randomCard(),randomCard(),randomCard());
returnthese;
}
publicvoidprintHand(Handh)
{
for(intc=0;c<5;c++)
{
printCard(h.cards[c]);
}
}
publicbooleancheckFlush(Handh)
{
if(h.cards[0].suith.cards[1].suit
&&h.cards[1].suith.cards[2].suit
&&h.cards[2].suith.cards[3].suit
&&h.cards[3].suith.cards[4].suit
)
{returntrue;}
else
{returnfalse;}
}
OVERVIEW
Poker Suits Line
- The player must place a wager on the main blackjack game first, and may wager on the optional sidebets.
- After all wager are placed, traditional blackjack is dealt.
- After the cards have been dealt, the side wagers are settled. If the player has an Ace in their hand, but does not have Blackjack, they are paid according to the “Player Any Ace” payout.
- If the player has two Aces of two Kings and the dealer’s up-card is an Ace or a King creating a Three of a Kind, they are paid according to the posted paytable.
- If the player has Blackjack, then one of the “Blackjack” pays will be paid out. The Blackjack Match wager pays according to the hosted paytables.
- Normal blackjack play will continue after Blackjack Match wagers are settled.
- Must Hit By: Blackjack Match can also feature a “Must Hit By” amount. This dollar amount, noted on the STAX progressive display, may be awarded at the end of the round to a player making the optional progressive bet.
HAND DEFINITIONS
- Ace/King Blackjacks Matching Suits: Player and dealer have A-K, all 4 cards of the same suit.
- Matching Suited Blackjacks: Player and dealer identical blackjacks, all 4 cards of the same suit.
- Three Aces: Player A-A and dealer up-card is Ace.
- Three Kings: Player K-K and dealer up-card is King.
- Player & Dealers Suited Blackjacks: Player suit does not need to match dealer suit.
- Player & Dealer Blackjacks: Both player and dealer two-card 21s.
- Player Suited Blackjack: Player-only two-card 21, suited.
- Player Blackjack: Player-only two-card 21.
- Player Any Ace (first two cards): Either or both player cards are an Ace.